Before you dive into programming the SpinWheel, first give this adventure a try to learn more about what the SpinWheel is capable of. We’ll start with some simple animations and end with some code that you’ll add to the SpinWheel itself in later adventures.
Welcome to the SpinWheel educational materials! This page is the first of many adventures and lessons we have written to empower you create exciting new programs on your SpinWheel. If you have never programmed before, we hope that this lesson will help you become more comfortable with looking at new code and figuring out what it might do. If you already have coding experience, then you may prefer jumping to the Initial Setup Guide and then continuing into the other adventures.
The SpinWheel
When you pulled the SpinWheel out of the box and turned it on for the first time, the first thing you may have noticed is its LEDs. As you toggle between the programs on the SpinWheel using the button on the back, you can see some of the many things that the SpinWheel can do. You can program the LEDs to make repeating patterns or respond to the motion of the device. You can also light up all the LEDs in one color. As you go through the adventures and lessons in our Field Guide, you will learn how these animations were written and become comfortable customizing it further.
Controlling the SpinWheel
Before you start programming your SpinWheel, we will start by experimenting with some virtual SpinWheels right here in your internet window. Do not worry at this point about understanding fully what every line of code does. We encourage you to experiment with changing the parameters and then observing what happens. This may feel uncomfortable at first, but keep trying and don’t be afraid if you don’t understand everything the first time you read it.
Turning on the Large LEDs
To start with, let’s try turning on all of the big LEDs. Using the sliders, you can change the colors on the virtual SpinWheel below:
0
0
0
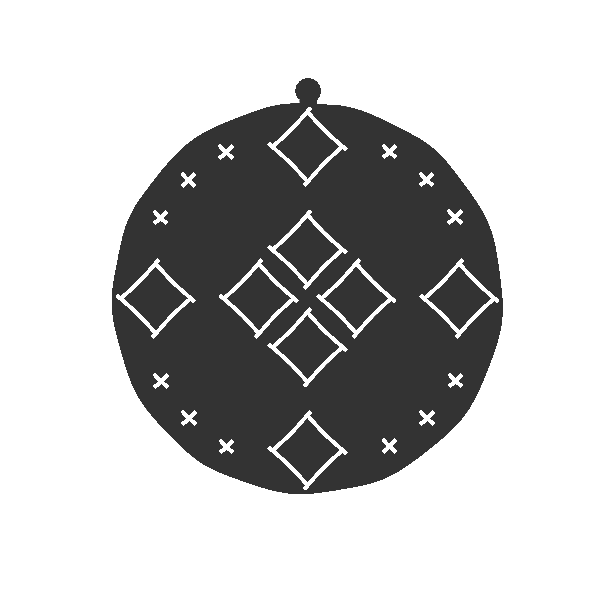
To learn why we are mixing colors using red, green, and blue,
check out our Biology of Sight adventure. On this page, you can try this out this on your own SpinWheel, as well as learn more about how our eyes work.
On your SpinWheel, rather than using sliders to change the color, you will instead use building blocks of code. On the virtual SpinWheel below, you can see how the code that you would run on your physical SpinWheel would change the LED display. For now, focus only on the line SpinWheel.setLargeLEDsUniform(50, 255, 250)
. It has three numbers that you can change to control the color of the SpinWheel LEDs. Like with the sliders, where you created colors by mixing red, green, and blue together, the first number, 50
, represents the amount of red, the next, 255
, represents the amount of green, and the final, 250
, gives the amount of blue. These combinations give a bright blue color. Press the “Run for 10 seconds” button to see what happens.
void loop() { SpinWheel.clearAllLEDs();
SpinWheel.drawFrame(); }
Try changing the code for yourself by typing the numbers you want instead of 50, 255, 250
in the line SpinWheel.setLargeLEDsUniform(50, 255, 250)
. Then, press “Run for 10 seconds” to see the color you created on the SpinWheel.
If you are really curious about what those other lines mean, we explain them more in our Basic Structure of a Program lesson. We recommend doing that page after the Initial Setup Guide.
It is also possible to specifically control each large LED. With the first slider, you can select the LED that you want to change the color of. Then, using the other sliders, you can adjust the color of that LED. Repeat this process for each LED you want to change the color of. If you move all three sliders for a given LED to zero, then that will turn the LED “off” again. Experiment with the LED number slider to see how the numbering of the LEDs works (or check out the diagram at the bottom of this section).
LED Number 0
255
255
255
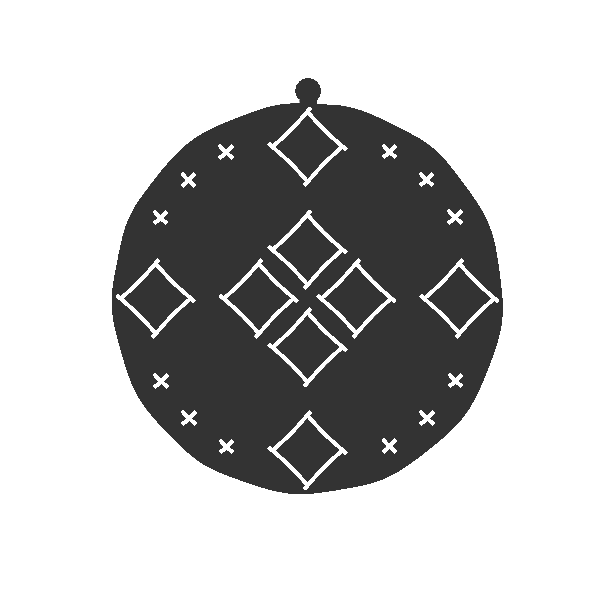
Now let’s try doing the same thing, but using the code you will put on your SpinWheel. To start with, we are just turning on one LED.
void loop() { SpinWheel.clearAllLEDs();
SpinWheel.drawFrame(); }
You can also copy this line and independently control each large LED. This is similar to how above you could change the color of each LED. Rather than adjusting sliders, copy the SpinWheel.setLargeLED
line and change the first number. For instance, to light up the very top large LED in the same color, add SpinWheel.setLarge(4, 255, 0, 100);
. The first number gives the LED and the next three give the amount of red, green, and blue light (like in the SpinWheel.setLargeLEDsUniform
command). Try adding more LEDs or changing the color yourself on the SpinWheel!
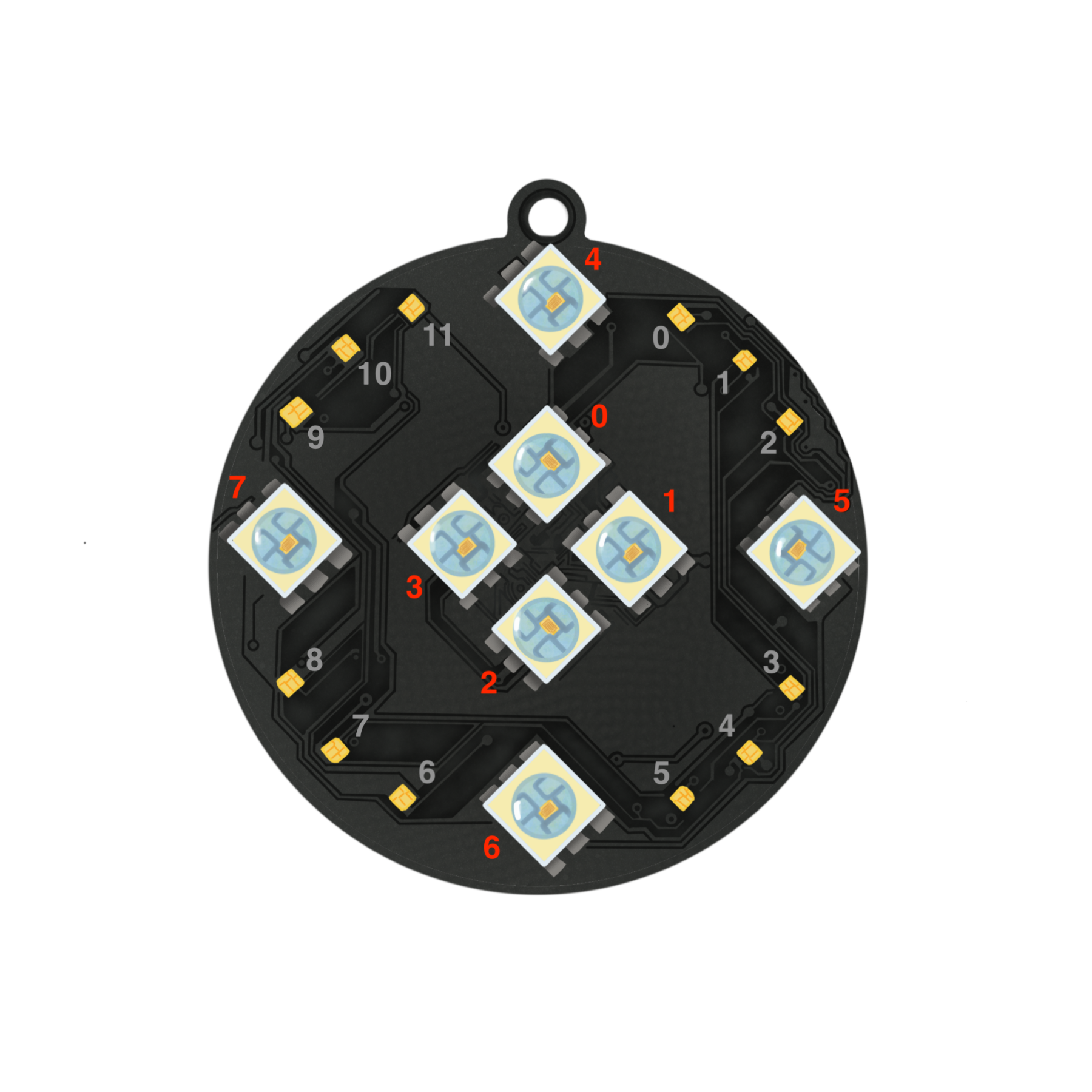
Turning on the Small LEDs
You can also turn on all of the small LEDs. To do this, instead of using SpinWheel.setLargeLEDsUniform
, you can use the line
SpinWheel.setSmallLEDsUniform
. Like above, the three numbers represent the red, green, and blue components of the color you want to make.
void loop() { SpinWheel.clearAllLEDs();
SpinWheel.drawFrame(); }
In the same way that you used SpinWheel.setLargeLED
, you can also use SpinWheel.setSmallLED
to control each of the 12 small LEDs on their own. Experiment with adding more lines to turn on more of the small LEDs or create a completely different pattern.
void loop() { SpinWheel.clearAllLEDs();
SpinWheel.drawFrame(); }
Controlling all of the LEDs
It is also possible to control each large and small LED independently, below we turn on all of the LEDs together:
void loop() { SpinWheel.clearAllLEDs();
SpinWheel.drawFrame(); }
At this point, you can modify the code above to create your own pattern that uses all of the LEDs. We encourage you to take some time to experiment with this to create your own designs. Once you have some patterns that you are excited to try on your own SpinWheel, proceed to the Initial Setup Guide that leads you through installing the computer program necessary to communicate with the SpinWheel. Then work through the Basic Structure of a Program lesson that will get you to the point where you can put the code that you wrote above onto your own SpinWheel.